Multi-Label Genre Classification-Part III
Deploying the saved movie classifier
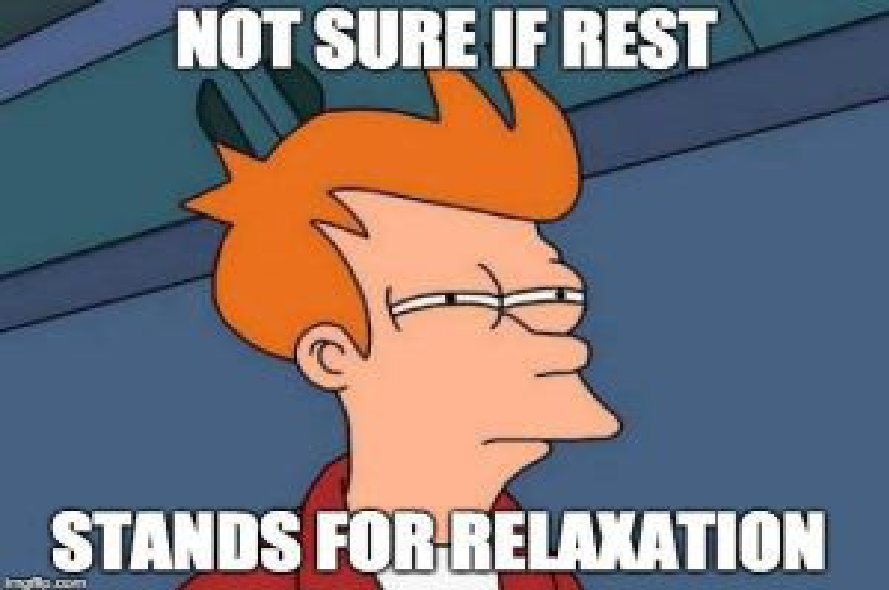
To create something people want
Deployment
Deploy the model as an API
import json
import tarfile
import pandas as pd
import re
import string
import os
from tqdm import tqdm
from pattern.text.en import singularize
from sklearn.model_selection import train_test_split
import tensorflow as tf
from transformers import BertTokenizerFast, TFBertModel
import numpy as np
import tempfile
import sys
import subprocess
from datetime import datetime
from packaging import version
import matplotlib.pyplot as plt
import seaborn as sns
%matplotlib inline
if 'google.colab' not in sys.modules:
SUDO_IF_NEEDED = 'sudo'
else:
SUDO_IF_NEEDED = ''
!echo "deb http://storage.googleapis.com/tensorflow-serving-apt stable tensorflow-model-server tensorflow-model-server-universal" | {SUDO_IF_NEEDED} tee /etc/apt/sources.list.d/tensorflow-serving.list && \
curl https://storage.googleapis.com/tensorflow-serving-apt/tensorflow-serving.release.pub.gpg | {SUDO_IF_NEEDED} apt-key add -
!{SUDO_IF_NEEDED} apt update
!{SUDO_IF_NEEDED} apt-get install tensorflow-model-server
os.environ["MODEL_DIR"] = <YOUR_MODEL_DIRECTORY>
%%bash --bg
nohup tensorflow_model_server \
--rest_api_port=8501 \
--model_name=picture_sniffer \
--model_base_path="$MODEL_DIR" >server.log 2>&1
def predict_genres(MAX_LEN, tokenizer):
while True:
user_inp = input("Enter the plot summary of the movie you wish to identify: ")
if user_inp == '':
break
print(user_inp)
print()
print('Genres:')
user_inp = [user_inp]
tokenized_data = tokenize_data(MAX_LEN, user_inp, tokenizer)
data = json.dumps({'signature_name':'serving_default',
'inputs': {'input_ids':tokenized_data[0].numpy().reshape(-1, 256).tolist(),
'attention_mask': tokenized_data[1].numpy().reshape(-1, 256).tolist()}})
headers = {'content-type':'application/json'}
json_response = requests.post('http://localhost:8501/v1/models/picture_sniffer:predict', data = data, headers = headers)
predictions = json.loads(json_response.text)['outputs']
predictions = np.squeeze(np.asarray(predictions))
labels = preprocessed_df.iloc[:, 3:].columns
maxm = max(predictions)
for i in range(len(labels)):
if maxm/predictions[i] <= 3:
print(f'{labels[i]}:{predictions[i]*100}%')
predict_genres(MAX_LEN, tokenizer)
Conclusion
In conclusion, the movie classification model presented here offers an effective and efficient solution for categorizing movies based on their genres. By leveraging advanced machine learning techniques and a comprehensive dataset, the model demonstrates a high level of accuracy in predicting genres for a given movie.